Overview
Seller Manager Lite is a desktop application used for managing a business operational and logistical needs. The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 28 kLoC. It was morph from the original Address-Book 3 application.
Summary of contributions
-
Major enhancement: added the export to csv feature
-
What it does: allows the user to export current data into excel readable CSV files. Using export command, user can easily export the current customer and phone data into CSV file available for printing.
-
Justification: This feature improves the product significantly because a user seller can easily create a CSV file containing the business data such as the inventory of phones and import into excel to print easily. This allows the seller to easily create a hard copy of the current inventory to do easy stock take in real life.
-
Highlights: I have written the command [Export] that reads present Json files to create JsonObjects. The creation of CSV file using JsonObject uses a third-party library to convert Json string into JsonObjects and then into JsonArray to be finally converted into CSV format string. To make the feature useful for the users, I have designed the printable CSV file to display just the necessary attributes so as to keep the customer list and phone inventory neat and easy to read.
-
Credits: Used org.json library to handle JsonObjects, JsonArrays and support comma delimiter to create CSV.
-
-
Other Major enhancement: Designed and implemented the Json saving feature that is used to save data of the different models in our application. The feature is inspired by AB3 use of Json saving feature. This is done by morphing present AB3 saving features to be integrated into our application.
-
Code contributed: [Reposense]
-
Other contributions:
-
Project management:
-
Managed releases
v1.3
-v1.5rc
(3 releases) on GitHub
-
-
Enhancements to existing features:
-
Documentation:
-
Community:
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Export data: export
Exports application data into csv file.
If csv file of the input file name exists, the application data will be exported to the existing file.
If csv file of the input file name does not exist, the application data will be exported into a new file.
-
Write the export command:
Export FILENAME
-
The CSV file of file name "FILENAME" will be created in the data folder.
Format: export FILE_NAME
Import data : import
[coming in v2.0]
Imports csv file into the application.
If csv file of the input file exists, the file will be imported.
If csv file of the input file does not exist, command will not be executed.
Format: import FILE_NAME
Export data to PDF [coming in v2.0]
Export the application data in SML to PDF format.
Format: export pdf
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Storage component
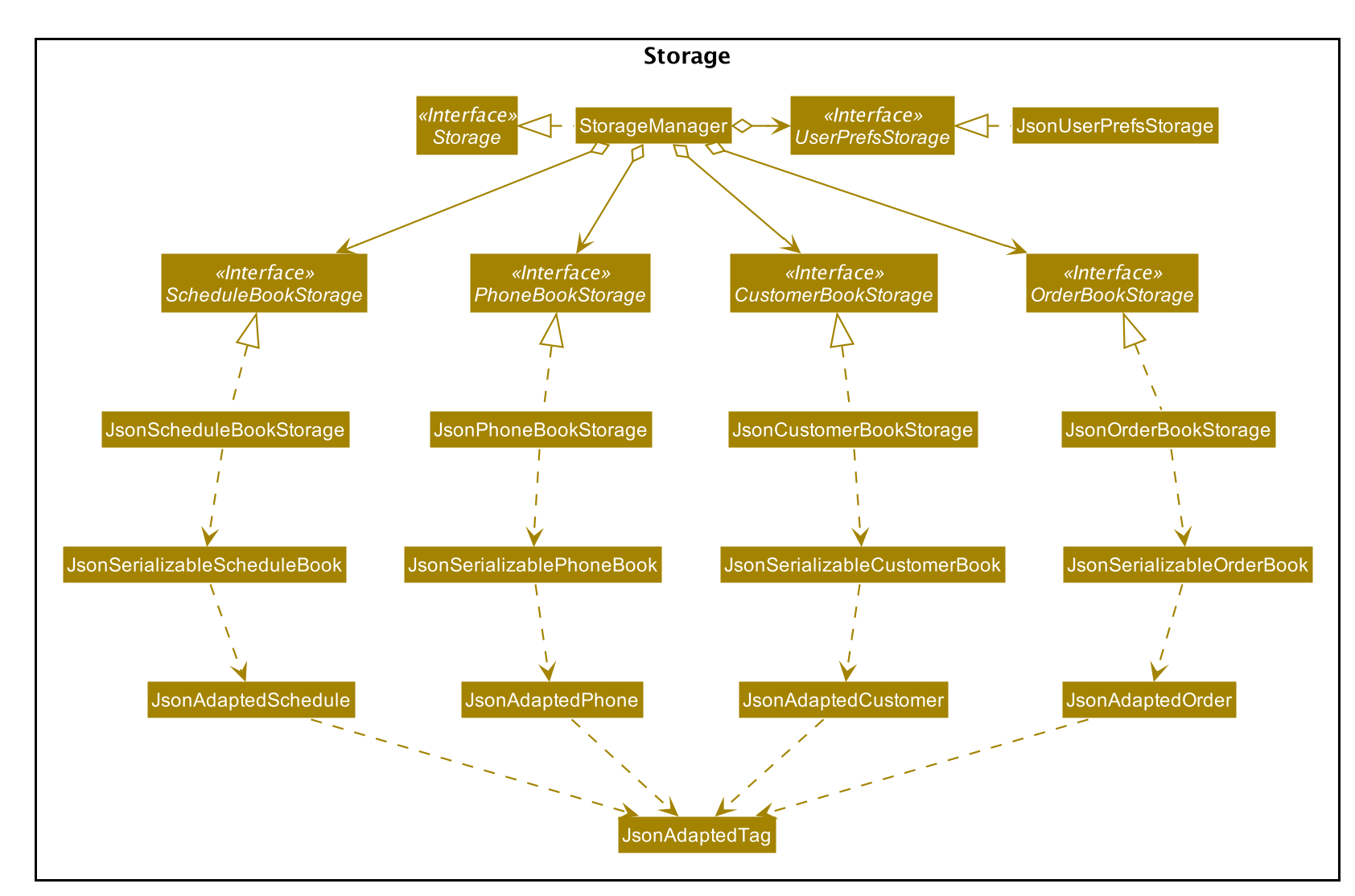
API: Storage.java
The Storage
component,
-
can save
UserPref
objects in JSON format and read it back. -
can save the Customer Book data, Phone Book data, Order Book data and Schedule Book data in JSON format and read them back.
Export feature
Implementation
The export mechanism is allows user to export current saved Json files into csv files available for import into MSExcel.
We made use of FileReader
to read the Json saved data files. The data is used to create JsonObjects
and stored into JsonArray
and then CSV formatted string using comma delimiters.
Additionally, it implements the following operations:
-
ExportCommand#getCSVString()
— Returns CSV formatted string containing the customer data, phone data, order data and schedule data.
How it works.
Given below is an example usage scenario and how the export mechanism behaves at each step.
The user executes export filename
command to export current data into a CSV file.
UserPrefs
is created and used to get the file path of the data files (i.e. customerbook.json).
The method getCSVString is executed to create FileReader
and BufferedReader
to read the data files from each of the path.
The string is then used to create JsonObject
and then converted to CSV formatted string data representation.
At the end, the strings are appended to get the full CSV file containing the various data.
Design Considerations
-
Alternative 1 (current choice): Converting Json formatted string into CSV formatted string.
-
Pros: Easy to implement.
-
Cons: Customisation to design CSV layout requires re-formatting of the string.
-
-
Alternative 2: Creating CSV formatted string straight from the Model.
-
Pros: Easier to implement on the fly the decided layout of created CSV file.
-
Cons: Harder to maintain. Had to code for the various cases in different data.
-
Current Implementation
Break down of the command.
Below is a more in-depth explanation at each step.
Step 1: User inputs a the export command e.g Export FILENAME
The commandBox executes it and the MainWindow runs its executeCommand(commandText) method. Referring to the sequence diagram below, this results in logic.execute(commandText) being called and the ExportParser parses the input from the user, returning a Command object.
Step 2: The logic then calls command.execute(command)
When this happens the ExportCommand
execute creates a new CommandResult object and return that to Logic, completing the execution of the user input command.
Step 3: The commandResult executes getCSVString()
which accesses JsonFiles in the data folder of Storage to parse the data into CSV strings and returns the data.
Step 4: The commandResult creates CSV file in data folder and returns in the end of execution.
Shown below is a quick summary of step 1-4 (Full complete diagram):
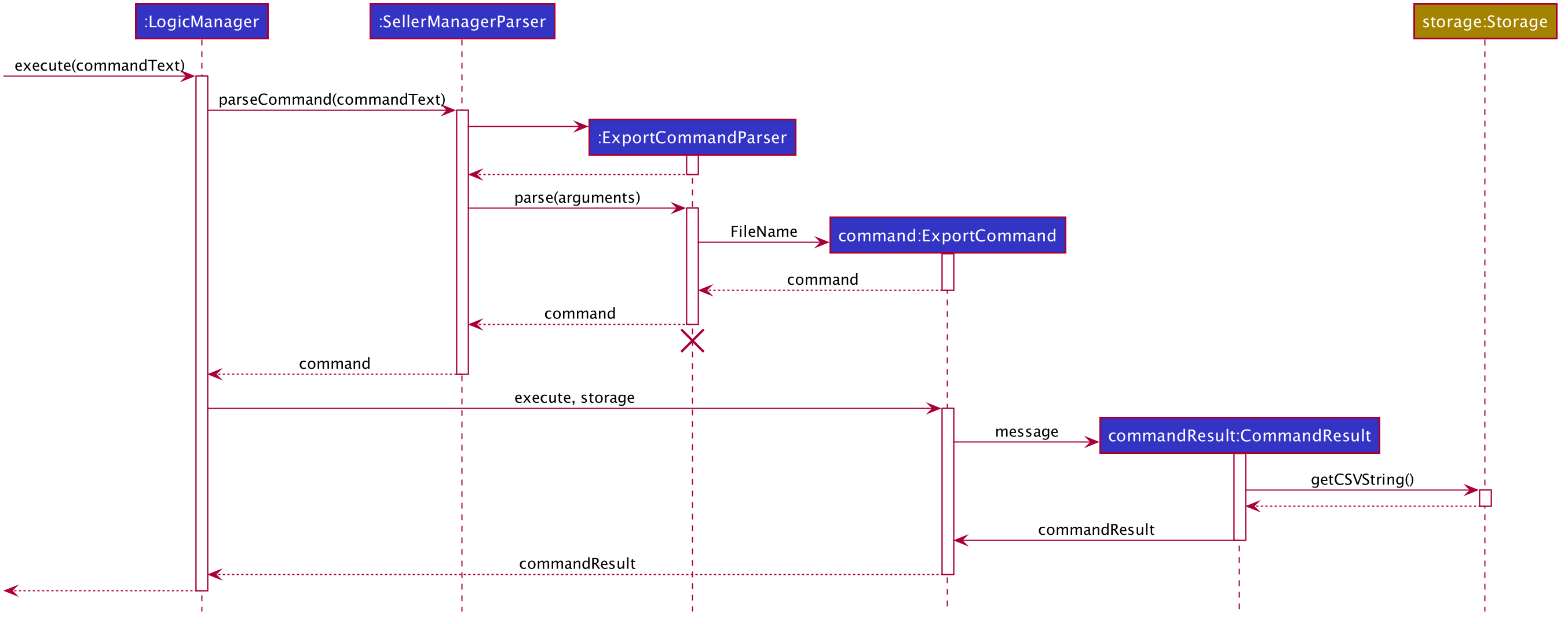
Notes to developer
Developers working on adding features to this module should take note of these limitations that we have put in place for v1.4
of SML:
-
File names are limited to the maximum character limit of Mac and Window users of 255 characters.
-
Simplified handling of file name which only allows file names to be alphanumeric.
Saving data
-
Data is automatically saved after every command.
-
If all files are missing, sample data files will be loaded.
-
Dependency flows from schedulebook to orderbook to phonebook and customerbook.
-
If file "a" has dependency on file "b", and file "a" is missing, both empty file "a" and "b" will be loaded.
-
If file is corrupted i.e. wrong string input, sample file will be loaded.
-