Overview
Seller Manager Lite (SML) is a desktop application used for handphone resellers to manage their customers, phones, orders and schedules all on one platform. The user interacts with it using a Command Line Interface (CLI), and it has a Graphical User Interface (GUI) created with JavaFX. It is written in Java, and has about 10+ kLoC.
Summary of contributions
-
Major enhancement: added GUI to display schedules in a calendar.
-
What it does: This feature allows our users to view their schedules graphically, as well as navigate around the panel after adding/editing/deleting schedules.
-
Justification: This feature is a critical addition for sellers who have numerous schedules planned throughout the week. Without a visual representation of a calendar, it would be extremely non-user-friendly as sellers would have to check through their list of schedules and the timings one by one.
-
Highlights: I designed a panel class to contain the third-party library for rendering events as a calendar. The class acts as a wrapper around the calendar and contains the logic for controlling the calendar. To make the application more user friendly, the application automatically switches to the calendar panel when any schedule commands are executed. This is to provide users a visual confirmation of the results of their command.
-
Credits: Used a third party library JFXtras in Calendar Panel to render schedules visually.
-
-
Other enhancement:
-
Schedule commands (Logic) to complement the calendar display.
-
Assisted to set up some parts in Model initially. (Order and Schedule)
-
-
Code contributed: RepoSense tP Code Dashboard
-
Other contributions:
-
Project management:
-
Added some issues in issue tracker. (Issues)
-
-
Documentation:
-
Community:
-
Tools:
-
Integrated a third party library (jfxtras.Agenda) into SML.
-
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Schedule Commands
Commands that work on schedules you have.
Switch to Schedule Tab Panel: switch-s
Switches to Schedule Tab Panel.
Format: switch-s
Go to a specific date in the calendar: schedule
Shows the week of the date specified by the user.
Format: schedule cd/DATE
Date should be in the format YYYY.MM.DD with valid year, month and date. Only dates from the year 1970 onwards are valid. |
Month and date can be single digits where applicable. |
Add a schedule: add-s
Adds a schedule.
If there are conflicts with the existing schedules, use the -allow
flag to allow clashing schedules.
Format: add-s ORDER_INDEX cd/DATE ct/TIME v/VENUE [t/TAG]… [-allow]
Example:
-
add-s 3 cd/2018.7.25 ct/18.00 v/Starbucks t/freebie -allow
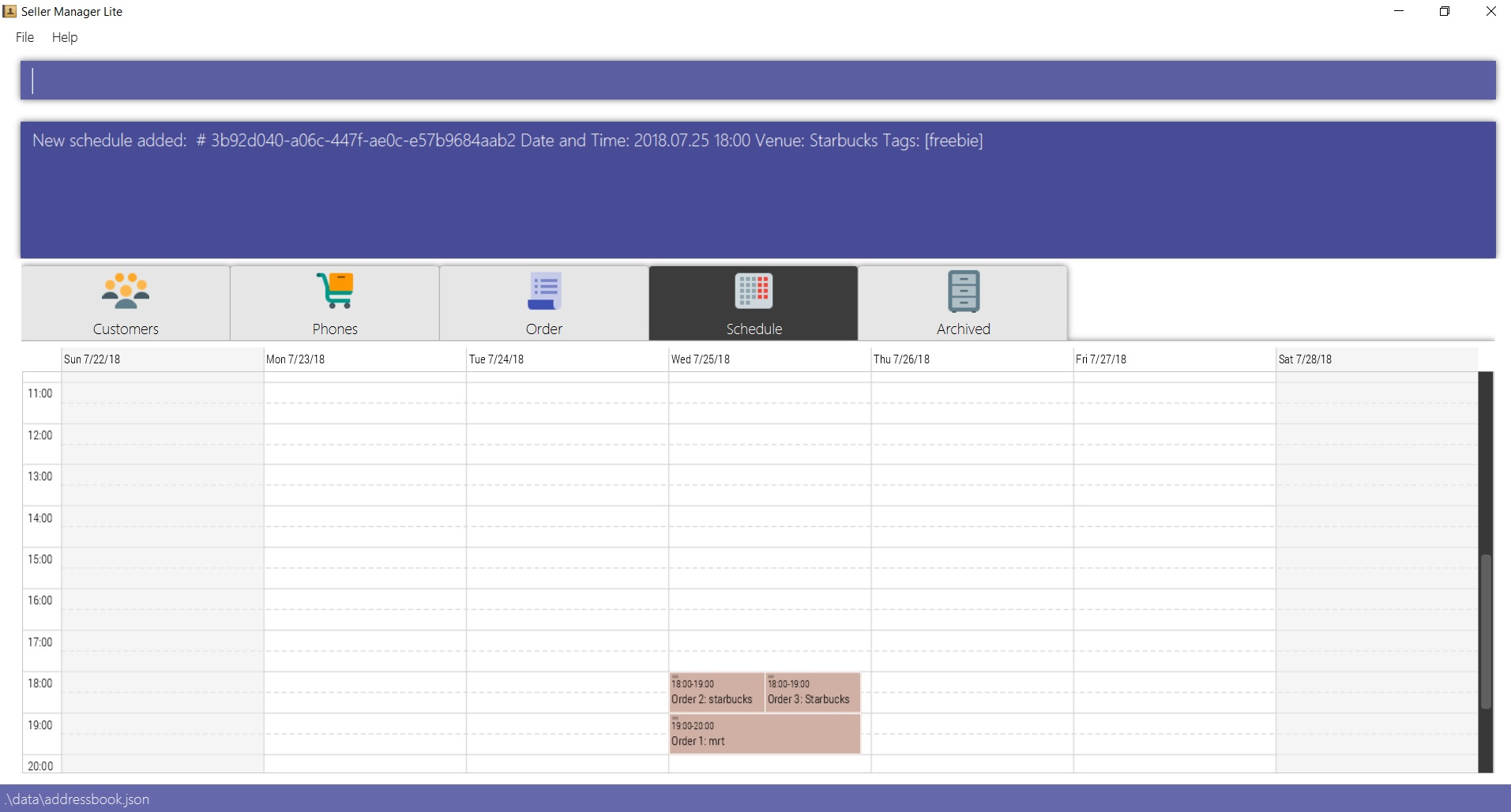
Order index should be a positive integer and must exist in the order list. |
Date should be in the format YYYY.MM.DD with valid year, month and date. Only dates from year 1970 onwards are valid. Month and date can be single digits if applicable. |
Time should be in the 24-hour format HH.MM with valid hour and minute. Hour and minute can be single digits if applicable. |
Schedule can have any number of tags, including 0. |
Delete a schedule: delete-s
Deletes a schedule.
Format: delete-s ORDER_INDEX
Example:
-
Delete the schedule of the 2nd order.
-
list-o
-
delete-s 2
-
Edit a schedule: edit-s
Edits an existing schedule.
If the edited schedule has conflicts with the existing schedules, use the -allow
flag to allow clashing schedules.
Note: You can add multiple schedules at the same time slot but it will affect the visibility of the order index and schedule venue on the calendar.
Format: edit-s ORDER_INDEX [cd/DATE] [ct/TIME] [v/VENUE] [t/TAG]… [-allow]
Examples:
-
Edit the date of the schedule of the 1st order and allow it to clash with the existing schedules.
edit-s 1 cd/2019.12.12 -allow
Customise schedule duration [coming in v2.0]
There will be an additional attribute in the schedule which takes in the duration of the event (in minutes).
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
-
Logic
uses theSellerManagerParser
class to parse the user command. -
This results in a
Command
object which is executed by theLogicManager
. -
The command execution can affect the
Model
(e.g. adding a customer/phone/order/schedule). -
The result of the command execution, a
String
message andUiChange
enum is encapsulated as aCommandResult
object which is passed back to theUi
. -
In addition, the
UiChange
enum in theCommandResult
object can also instruct theUi
to perform certain actions, such as displaying the respective panels or help/statistics window.
Given below is the Sequence Diagram for interactions within the Logic
component for the execute("delete-c 1")
API call.
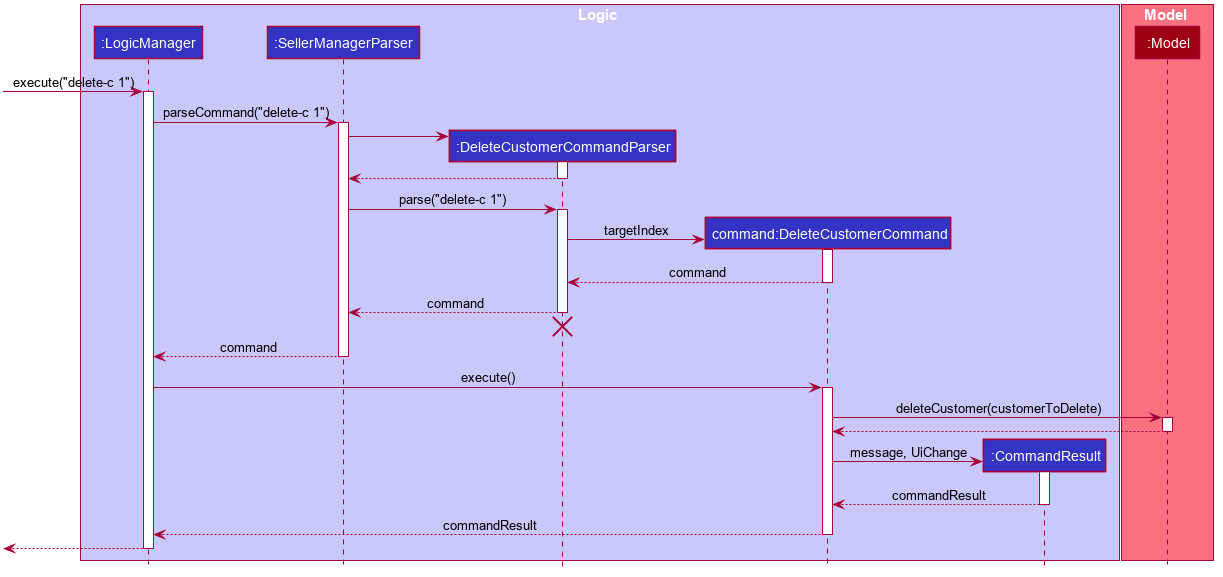
delete-c 1
Command
The lifeline for DeleteCustomerCommandParser should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of diagram.
|
Calendar / Schedule feature
Implementation
Overview
We decided to incorporate a third-party library, JFXtras’s Agenda, to represent our schedules on a calendar for easy viewing by our users.
The full documentation for Agenda
can be found here.
Calendar Panel
CalendarPanel
is a wrapper class designed to contain Agenda
for rendering events as a calendar.
Here is the class diagram for CalendarPanel
:
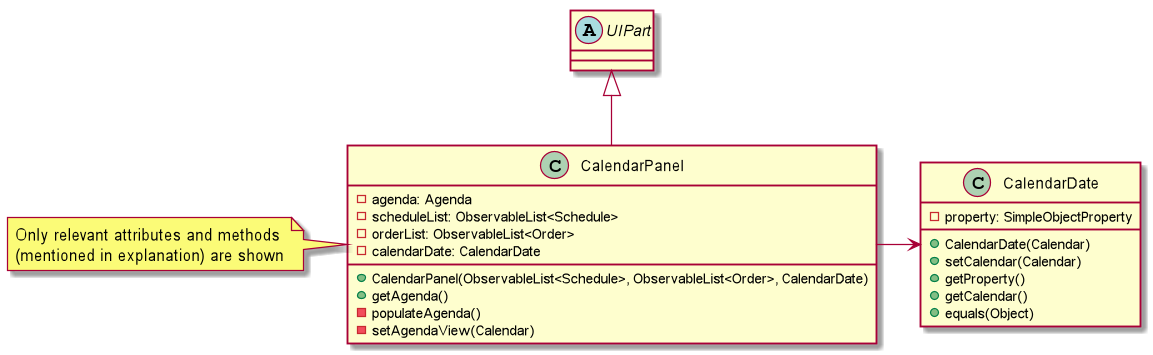
Integration with SML
-
On instantiation of
CalendarPanel
, all the schedules in scheduleList will be converted toAppointments
and then added toAgenda
viapopulateAgenda()
. -
To set the display information of the
Schedule
object, we will find itsOrder
in the orderList and retrieve its index. -
Date shown on the calendar will be today’s date by default.
-
Adds a listener to
scheduleList
. -
Adds a listener to
calendarDate
.
Complementing CRUD commands
SML supports a few main Schedule
commands:
-
Add — adds a new schedule into SML, command will be in this format:
add-s ORDER_INDEX cd/DATE ct/TIME v/VENUE [t/TAGS] [-allow]
-
Edit — edits an existing schedule in SML, command will be in this format:
edit-s ORDER_INDEX [cd/DATE] [ct/TIME] [v/VENUE] [t/TAGS] [-allow]
-
Delete — deletes an existing schedule in SML, command will be in this format:
delete-s ORDER_INDEX
-
Schedule — switches the view on the panel to the week containing the date entered, command will be in this format:
schedule cd/DATE
How the display works
The schedules and date in the calendar panel shown are automatically updated depending on the commands executed by the users. For example:
-
If the user adds/edits/deletes a schedule — calendar panel will display the week with the date of the schedule.
-
If the user uses the switch-s command — calendar panel will display the week with today’s date.
-
If the user uses the schedule command — calendar panel will display the week with the date requested by the user.
Changes to the schedules are made in the Model
by editing its scheduleList.
CalendarPanel
listens to the changes and calls populateAgenda()
to generate the updated list of Schedules
into Appointments
to add to Agenda
.
The current date shown on the calendar panel is encapsulated in a CalendarDate
object.
The CalendarDate
class uses SimpleObjectProperty
to keep track of the Calendar (date) to be displayed.
When any of the schedule commands are executed, the model will edit CalendarDate
with the updated Calendar (date).
CalendarPanel
listens to the changes and calls setAgendaView()
to set the new Calendar
in CalendarDate
accordingly.
Here is an example of what happens when a user adds a schedule (AddScheduleCommand):
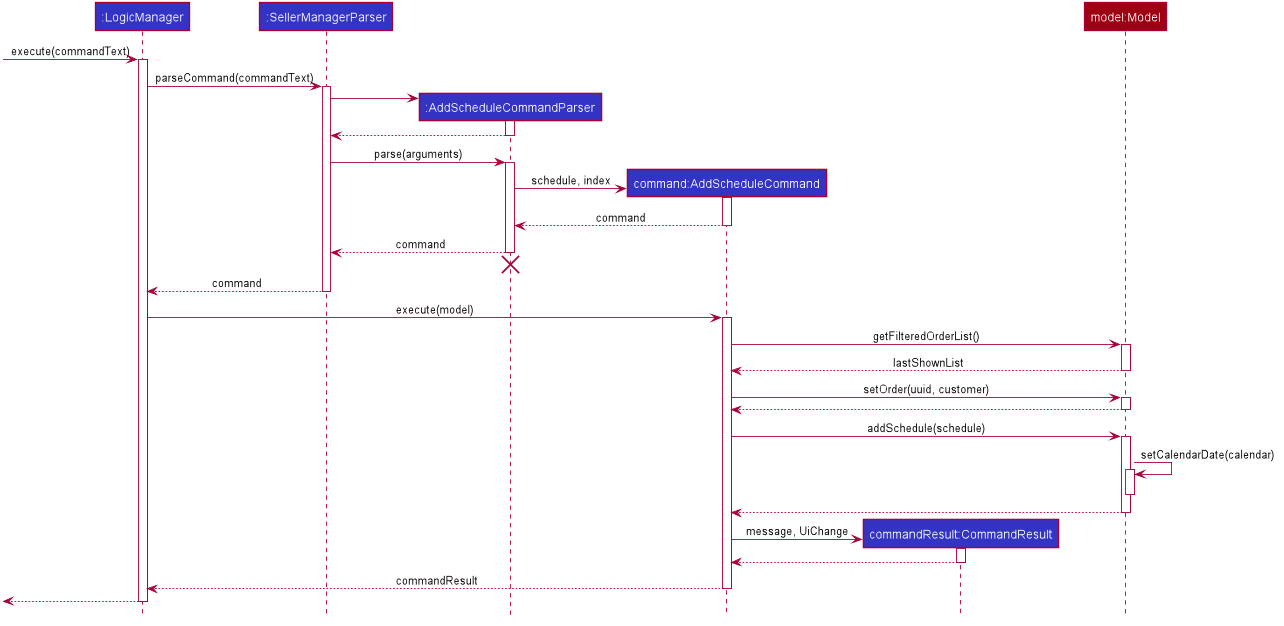
Basically, after the setCalendarDate()
method in Model
is executed, CalendarPanel
listens to it and makes the necessary changes to the agenda view by calling its setAgendaView()
method.
Design Considerations / Alternative designs considered
-
Whether schedules should be kept as
Appointment
objects instead ofSchedule
objects.-
We felt that the existence of our own
Schedule
object ensures that we can design it the way we want. -
We decided to not keep a list of
Appointment
inCalendarPanel
asAppointment
is only used forAgenda
while the rest of our code usesSchedule
. -
Instead, we have decided to only convert
Schedule
intoAppointment
when needed - during the creation ofCalendarPanel
or when there are changes made to theUniqueList<Schedule>
variable. Not keeping a list ofAppointment
ensures that we do not keep duplicate information.
-
-
Where to store and change the date shown on Calendar Panel.
-
The changes to
CalendarDate
was initially done in theCalendarPanel
itself. So,CommandResult
was made to have one more variableOptional<Calendar>
. In that case, every time a schedule command was executed, a specificCalendar
(if any) will be placed inside the constructor while creating theCommandResult
object. Else, Optional.Empty() will be placed inside. -
When the
MainWindow
executes the method performUiChanges(CommandResult), it will call the handleSchedule method withCalendar
(from CommandResult) as an argument. IfOptional<Calendar>
inCommandResult
is empty, today’s date and time will be used instead. Then,CalendarPanel
will be called in handleSchedule() to set the agenda view. -
This implementation was however changed because we thought that the date shown on the panel should be updated automatically. Hence, we decided to use a listener instead.
CalendarDate
is then placed inModel
so that it can be edited immediately whenModel
executes any schedule-related commands. Also, this ensures thatCommandResult
remains unchanged and kept as simple as possible.
-